Remember the times you knew alot about unit testing but would never think of actually seeing your precious models as units? Well, times changed and nowadays I really enjoy the good feeling of knowing that a 100% BPM testcoverage is relatively easy to achieve.
A very developer friendly way to create tests for process models is brought to us by Martin Schimak, with camunda bpm assert scenario:
"This community extension to Camunda BPM enables you to write robust test suites for process models. The larger your process models, the more often you change them, the more value you will get out of using it."
Check it out! You wont regret it.
The idea of a scenario test though is to really create an end-to-end scenario including execution of all sub processes. But sometimes I dont need or want this - so if there is one thing I would add to this project it's the ability to mock call activities. Hence seeing only the main process as unit under test.
But you can achieve this mocking anyway by simply deploying a mock-process model on the fly, no xml file needed, using Camundas BPMN model (fluent) api. Check this out:
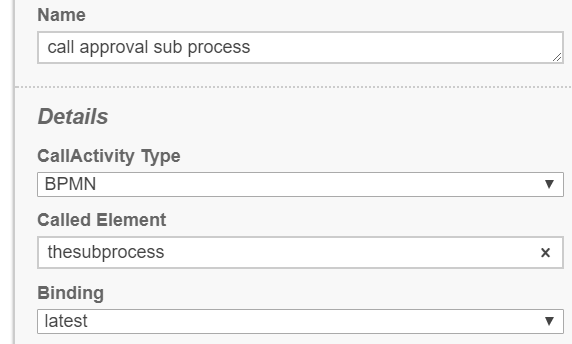
Lets pretend this would be our process under test and we are not interested in taking care of the called subprocess. We just want to mock it and its behaviour. All we want is to test, if the main process works.
We run a testcase like this:
@Test
public void test_rejection() {
deployMockedSubProcess("reject");
Scenario scenario = Scenario.run(process).startByKey("process").execute();
ProcessEngineAssertions.assertThat(scenario.instance(process)).hasPassed("EndEvent_Rejected");
}
As you can see, before we actually kick off the process, we deploy a lightweight process model on the fly which will produce a mocked outcome. In this case we set the outcome to reject. Note: The id of this mock has to be the same id the main process is loocking for. In our case it is thesubprocess.
Here is the method:
private void deployMockedSubProcess(String result) {
BpmnModelInstance modelInstance = Bpmn.createExecutableProcess() //
.id("thesubprocess") //
.startEvent() //
.serviceTask().camundaResultVariable("result").camundaExpression(result) //
.endEvent() //
.done();
Deployment deployment =
rule.getProcessEngine().getRepositoryService().createDeployment()
.addModelInstance("thesubprocess" + ".bpmn", modelInstance).deploy();
}
In alot of cases this really suffices and it is a handy way to getting things done if you do not want to go full scenario.
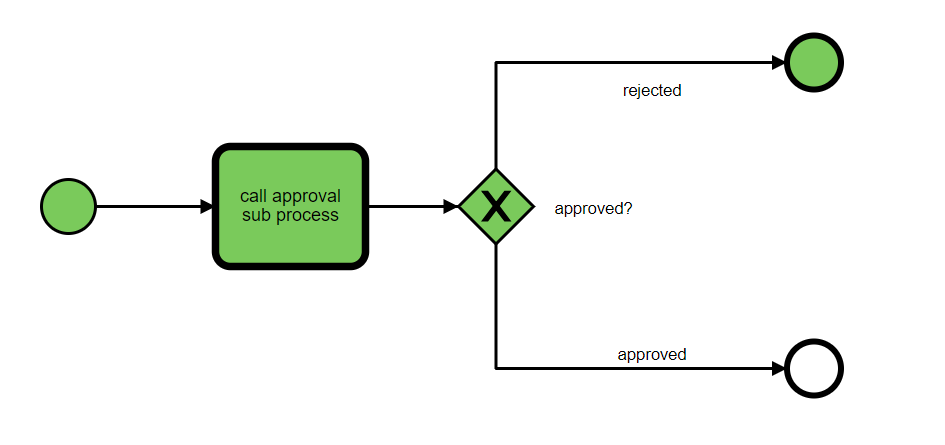
check out and run the code
https://github.com/stefanfrena/camunda/tree/master/assert-scenario-mock-call-activity
Let me know what you think and if I can help you to understand this better. This was just a rough nutshell.